Introduction
LibrePilot provides header files to use in an arduino programming environment. Using these header files, arduinos can read telemetry data via serial connection, as well as send commands via UAVTalk.
UAVObjectGenerator
Currently arduino support is available in the "next" branch: https://bitbucket.org/librepilot/librepilot/branch/next
You need to set up a build environment: Developer Manual
In your build environment, use the following commands to generate and show the header files:
make uavobjects_arduino
cd build/uavobject-synthetics/arduino/
ls -alh
LibrePilotSerial Library
A very small library makes it easy to access the objects in the telemetry stream.
The library is currently located at https://github.com/MarcProe/LibrePilot.arduino .
It is not mandatory to use this library, but it gives a good example for how to use the header files.
Currently supported functions:
- Request Object
(void request(unsigned long objId);
) - Receive Object (
boolean receive(unsigned long objId, byte *ret, unsigned int timeout = 100);
) - Send Object (
void send(unsigned long objId, byte* data, int length);
)
Todo:
- Handshake
- Receive multiple Objects (one at a time)
- ...
Connect to a flight controller
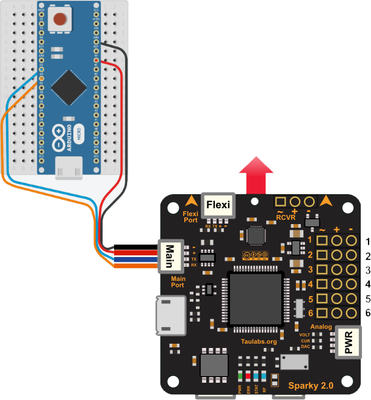
Mainport and Flexiport can be used to connect an arduino to and make use of serial communication.
Wire | Port | Arduino |
---|
Black | Ground | Ground |
Red | VCC | 5V |
Blue | Tx | Rx |
Orange | Rx | Tx |
Examples
Simple Example
//Library to use pins as serial port
#include <SoftwareSerial.h>
//Include a librepilot header file
#include "flightstatus.h"
//Include the LibrePilotSerial Library
#include "LibrePilotSerial.h"
//Initialize serial port
SoftwareSerial mySerial(2, 3); // RX, TX
//Initialize LibrePilot serial connection
LibrePilotSerial lps(&mySerial);
void setup() {
//Begin LibrePilotSerial communication
lps.serial->begin(57600);
}
void loop() {
//Request object from FC
lps.request(FLIGHTSTATUS_OBJID);
//Receive object from FC. This function will block until the specified object was received or it times out.
//It returns true if a valid packet was received
//the packet is stored in the array of the object packet union
boolean ok = lps.receive(FLIGHTSTATUS_OBJID, FlightStatusDataUnion.arr, 200);
//the packet data may only be accessed if the return value was true
if(ok) {
//the packet can be accessed in a structured manner via the data member
if (FlightStatusDataUnion.data.Armed == FLIGHTSTATUS_ARMED_ARMED) {
//the quad is armed, do something!
}
}
delay(250); //wait 250 ms
}
Arming LED
This sketch will read the FlightStatus.Armed
field from the flight controller and show the arming state on the RGB LED. Green for Disarmed, blue for Arming, red for Armed.
Curcuit:

(See on curcuits.io for BOM)
Sketch:
#include <SoftwareSerial.h>
#include "FlightStatus.h"
#include "LibrePilotSerial.h"
SoftwareSerial mySerial(2, 3); // RX, TX
LibrePilotSerial lps(&mySerial);
int ledPinr = 9;
int ledPing = 8;
int ledPinb = 7;
void setup() {
// initialize serial monitor:
Serial.begin(57600);
//initialize LibrePilotSerial Object
lps.serial->begin(57600);
}
void loop() {
lps.request(FLIGHTSTATUS_OBJID); //request object
//watch the telemetry stream for 200ms.
//Store object if it is received and return true. If not, return false.
boolean ok = lps.receive(FLIGHTSTATUS_OBJID, FlightStatusDataUnion.arr, 200);
Serial.print(" Result fs: ");
Serial.println(FlightStatusDataUnion.data.Armed);
if(ok) {
if(FlightStatusDataUnion.data.Armed == FLIGHTSTATUS_ARMED_DISARMED) {
analogWrite(ledPinr, 0);
analogWrite(ledPing, 255);
analogWrite(ledPinb, 0);
} else if (FlightStatusDataUnion.data.Armed == FLIGHTSTATUS_ARMED_ARMING) {
analogWrite(ledPinr, 0);
analogWrite(ledPing, 0);
analogWrite(ledPinb, 255);
} else if (FlightStatusDataUnion.data.Armed == FLIGHTSTATUS_ARMED_ARMED) {
analogWrite(ledPinr, 255);
analogWrite(ledPing, 0);
analogWrite(ledPinb, 0);
}
}
delay(500);
}
Related articles
-
Page:
-
Page:
-
Page:
-
Page:
-
Page: